What is React JS ?
React.js, commonly known as React, is a JavaScript library for building user interfaces. It was developed by Facebook and is widely used for creating dynamic, single-page applications where fast and efficient updates to the user interface are crucial. React allows developers to build reusable UI components and manage the state of an application in a more efficient and predictable way.
Why React JS ?
Its popularity stems from features and advantages designed to address common challenges in web development. Here are key reasons why React.js is favored:
Component-Based Architecture: React advocates for a component-based approach to constructing user interfaces. This strategy breaks down UIs into reusable, independent components, fostering modular, maintainable, and scalable code.
Virtual DOM: React’s utilization of the Virtual DOM enables efficient updates to the actual DOM. By updating a virtual representation and selectively modifying the necessary parts of the real DOM, React achieves enhanced performance and a smoother user experience.
Declarative Syntax with JSX: React employs a declarative syntax, where developers articulate the desired UI, and React manages the DOM updates accordingly. JSX, a JavaScript syntax extension, enhances code readability and expressiveness by allowing HTML-like code within JavaScript files.
Unidirectional Data Flow: React follows a unidirectional data flow, simplifying the understanding of data changes as they propagate through the application. Data flows from parent to child components, ensuring changes in child components don’t directly impact their parents.
React Native for Mobile Development: React Native, an extension of React, empowers developers to use React principles in creating native mobile applications for iOS and Android. This promotes code reuse between web and mobile applications, reducing development effort.
Large and Active Community: React boasts a sizable and engaged community of developers, providing abundant resources, tutorials, and third-party libraries. The active community support ensures React remains aligned with the latest web development best practices and trends.
Backed by Facebook: React is developed and maintained by Facebook, providing a foundation for continuous improvements and updates. The support of a major tech company instills confidence in the library’s long-term viability.
Flexibility and Compatibility: React’s flexibility allows integration with other libraries and frameworks, making it adaptable to various project requirements. It can be combined with tools like Webpack and Babel to build efficient and modern web applications.
How to setup React JS ?
Prerequisite
Well! There are some prerequisite before getting started with React Js Development.
- Node.js and npm (official Node.js website)
- IDE / Editor tool.
I will recommend Visual Studio Code (official Visual Studio Code Download)
Create React App
Open your terminal or command prompt.
Navigate to the directory under which you want to create your react app.
Use the following command to create a new React app using Create React App (a tool maintained by Facebook for easily setting up a new React project):
npx create-react-app hello-react-app
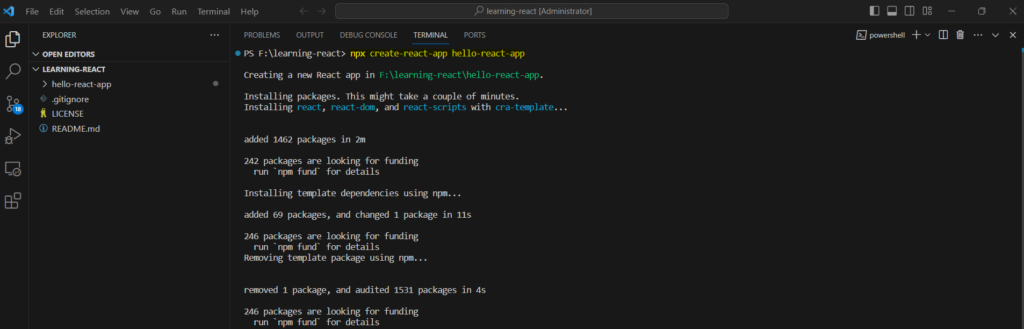
NOTE: I am using visual Studio Code Terminal to execute the Command.
Open Project
Open “hello-react-app” project folder in Visual Studio Code
Edit App.js
Replace Code in App.js with below code:
import './App.css';
function App() {
return (
<div className="App">
<header className="App-header">
<h1> Hello React App! </h1>
</header>
</div>
);
}
export default App;
Navigate to your Project in terminal
Run “cd” command in terminal window and navigate to project which was created in previous step.

Run the Development Server
Start the development server with the following command:
npm start

This will start the development server and open your React app in a new browser window. By default, the app runs on http://localhost:3000/
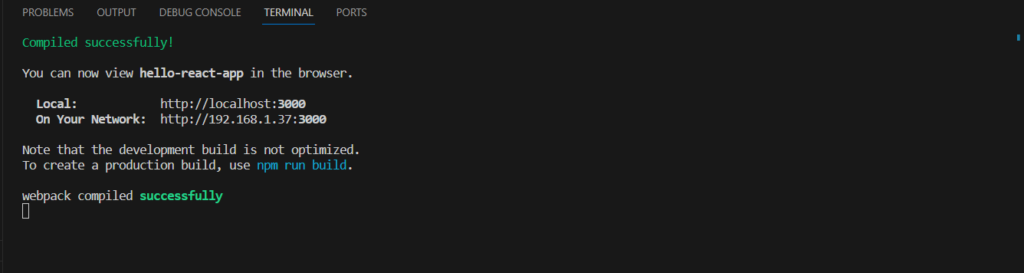
In your favourite browser you can navigate to http://localhost:3000/
and your react app is up and running.
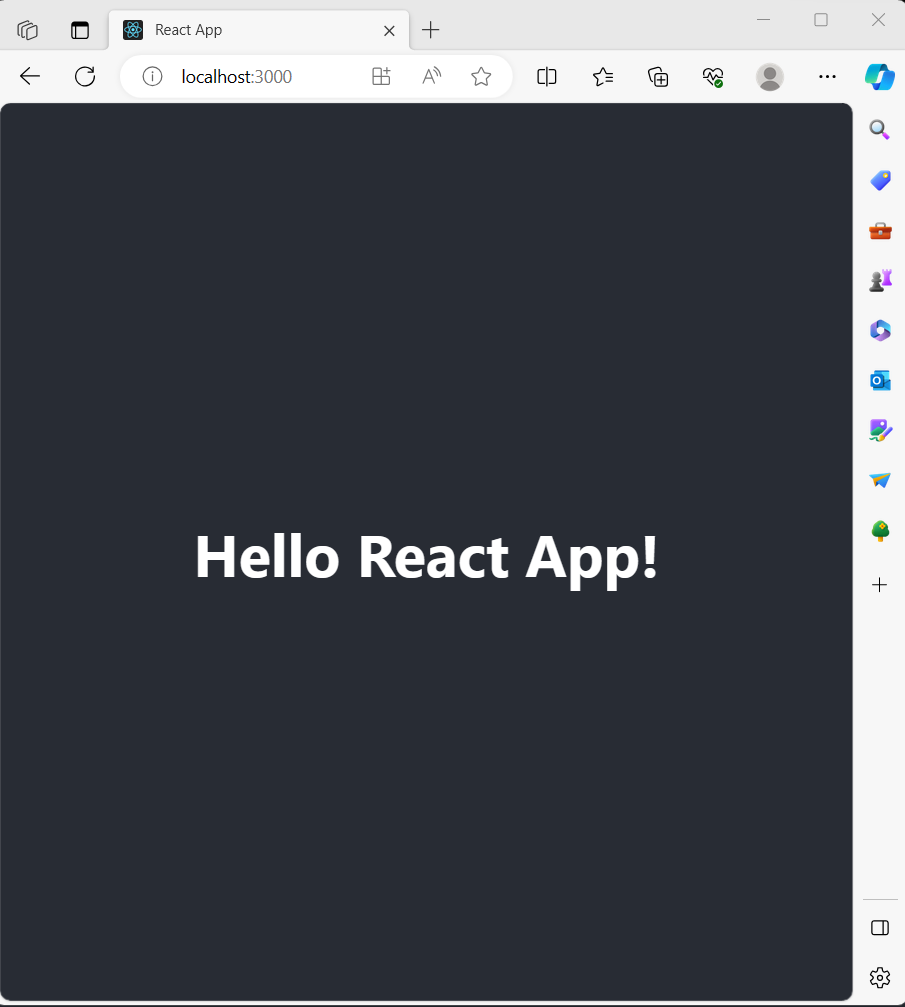
Conclusion
In this article we covered What, Why and How part of React Js App. More detailed explaination of project structure will be covered in another article.